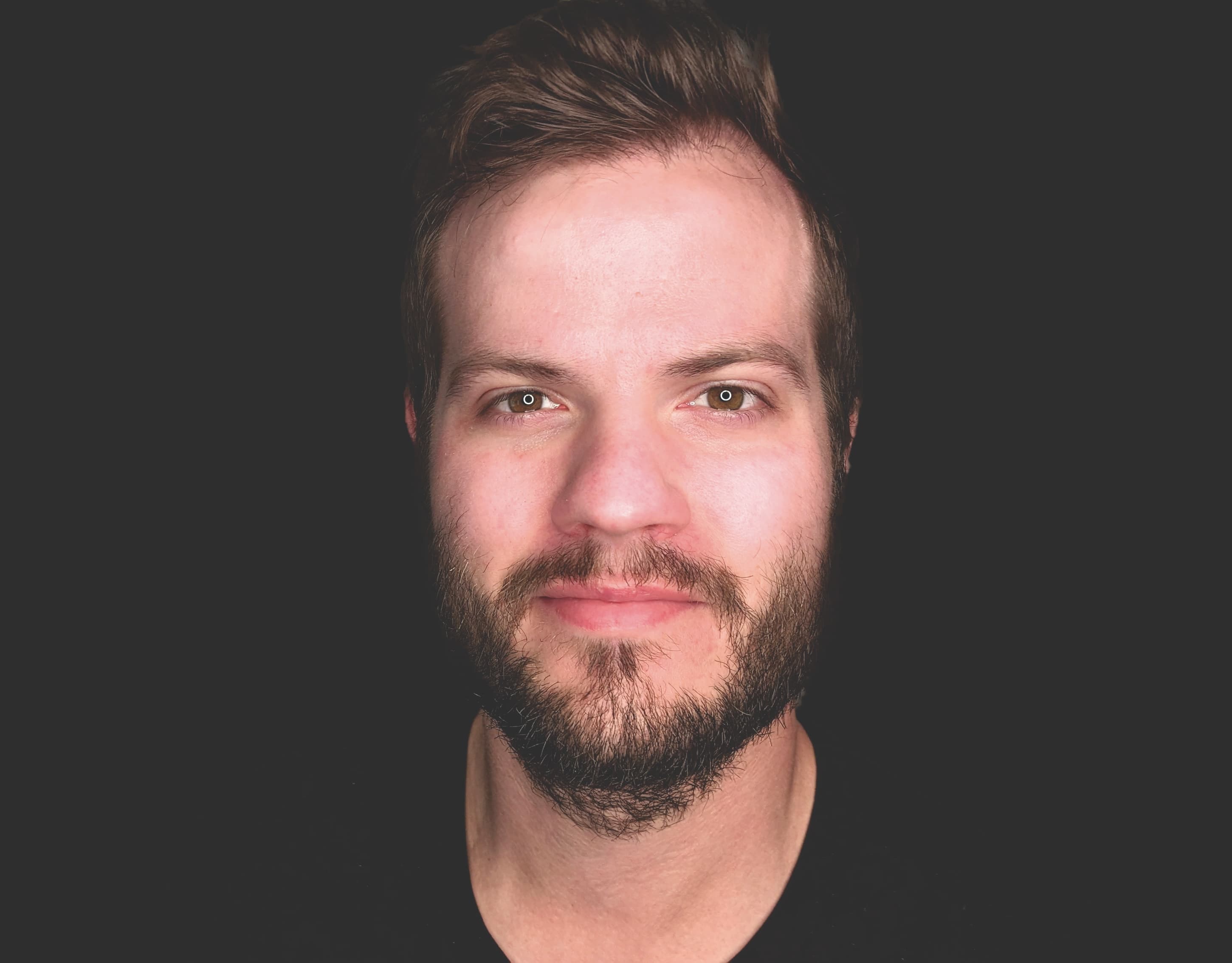
July 19, 2024
•Last updated July 19, 2024
ES6 Object-Oriented JavaScript Countdown Tutorial
In this tutorial, we will create a simple, reusable countdown widget using ES6 classes in JavaScript. The widget will accept options as parameters, allowing for flexibility in its use. This widget will be ideal for scenarios where you want to implement a countdown timer, such as for a game, a quiz, or any time-bound activity on your website.
The Countdown Class
Start by defining the Countdown class. I’ll leverage ES6 syntax and use its class and constructor patterns to make things more object-oriented.
class Countdown {
constructor(selector, options) {
this.element = document.querySelector(selector);
this.options = options;
this.intervalID = null;
this.remainingTime = this.options.seconds;
}
}
This class has a constructor that takes two parameters: a selector for the HTML element where the countdown will be displayed and an options object containing the initial countdown time in seconds.
The Start Method
Next, add the start
method to begin the countdown. We’ll source this method to kick off the logic shortly.
start() {
this.intervalID = setInterval(() => {
this.remainingTime--;
this.update();
if (this.remainingTime <= 0) {
this.stop();
}
}, 1000);
}
This method sets an interval that decreases the remaining time by one every second, updates the countdown display, and stops the countdown once the remaining time reaches zero.
The Stop Method
The stop
method is used to clear the interval and stop the countdown:
stop() {
clearInterval(this.intervalID);
this.intervalID = null;
}
The Update Method
The update
method is used to update the countdown display on the HTML element:
update() {
this.element.textContent = this.remainingTime;
}
Using the JavaScript Countdown
Now, you can create a new countdown as follows:
let countdown = new Countdown('#myCountdown', { seconds: 60 });
countdown.start();
This will create a 60-second countdown on the element with the ID myCountdown
. The countdown will start immediately, but there’s an opportunity to expand it to start after some optional delay.
You can use this countdown widget in any part of your website by creating a new instance of the Countdown class and simply passing the HTML element selector where you want to display the countdown, along with an object containing the initial countdown time.
Extending the JavaScript Countdown
The beauty of using ES6 classes is extending this basic Countdown class to add more functionality. For example, you might want to add a 'pause' feature to your countdown. To achieve this, you can extend the Countdown class and add a pause
method.
class CountdownWithPause extends Countdown {
constructor(selector, options) {
super(selector, options);
this.isPaused = false;
}
pause() {
if (!this.isPaused) {
clearInterval(this.intervalID);
this.isPaused = true;
}
}
resume() {
if (this.isPaused) {
this.start();
this.isPaused = false;
}
}
}
In the CountdownWithPause
class, we added a pause
method that stops the interval if the countdown is not already paused. We also added a resume
method that restarts the countdown if paused. This class inherits from the parent class it extends. Just remember to call the super
method within its constructor.
Use this extended countdown class as follows:
let countdown = new CountdownWithPause('#myCountdown', { seconds: 60 });
countdown.start();
// After some time pause
setTimeout(() => {
countdown.pause();
}, 1000)
// After some more time resume
setTimeout(() => {
countdown.resume();
}, 1000)
This will create a 60-second countdown on the element with the ID myCountdown
. The countdown will start immediately and can be paused and resumed at any time. For example, you might pause it if an action is taken.
Adding a Complete Callback to the JavaScript Countdown
Sometimes, you might want to perform some action when the countdown completes. This can be achieved by adding a complete
callback to our Countdown class. Of course, you can name anything you prefer.
class Countdown {
constructor(selector, options) {
//...
this.onComplete = options.onComplete;
}
//...
stop() {
clearInterval(this.intervalID);
this.intervalID = null;
if (this.onComplete) {
this.onComplete();
}
}
}
In the updated stop
method, we check if an onComplete
callback was provided in the options, and if so, we call it when the countdown stops.
To use this feature, you can pass a callback function in the options like so:
let countdown = new Countdown('#myCountdown', { seconds: 60, onComplete: function() {
console.log('Countdown Complete!');
}});
countdown.start();
In this example, "Countdown Complete!" will be logged to the console when the countdown reaches zero.
Another way to write this might be the following. It boils down to preference.
let countdown = new Countdown('#myCountdown', { seconds: 60 })
countdown.onComplete(() => {
// Do something
})
Conclusion
Using ES6 classes, we've created a flexible, reusable countdown widget in JavaScript. We've also seen how to extend our Countdown class to add additional functionality like pause and resume methods and include a completion callback. This demonstrates the power and versatility of object-oriented programming in JavaScript, enabling you to create complex, reusable components with ease.
Categories
Collection
Part of the JavaScript collection
Products and courses
-
Hello Hotwire
A course on Hotwire + Ruby on Rails.
-
Hello Rails
A course for newcomers to Ruby on Rails.
-
Rails UI
UI templates and components for Rails.