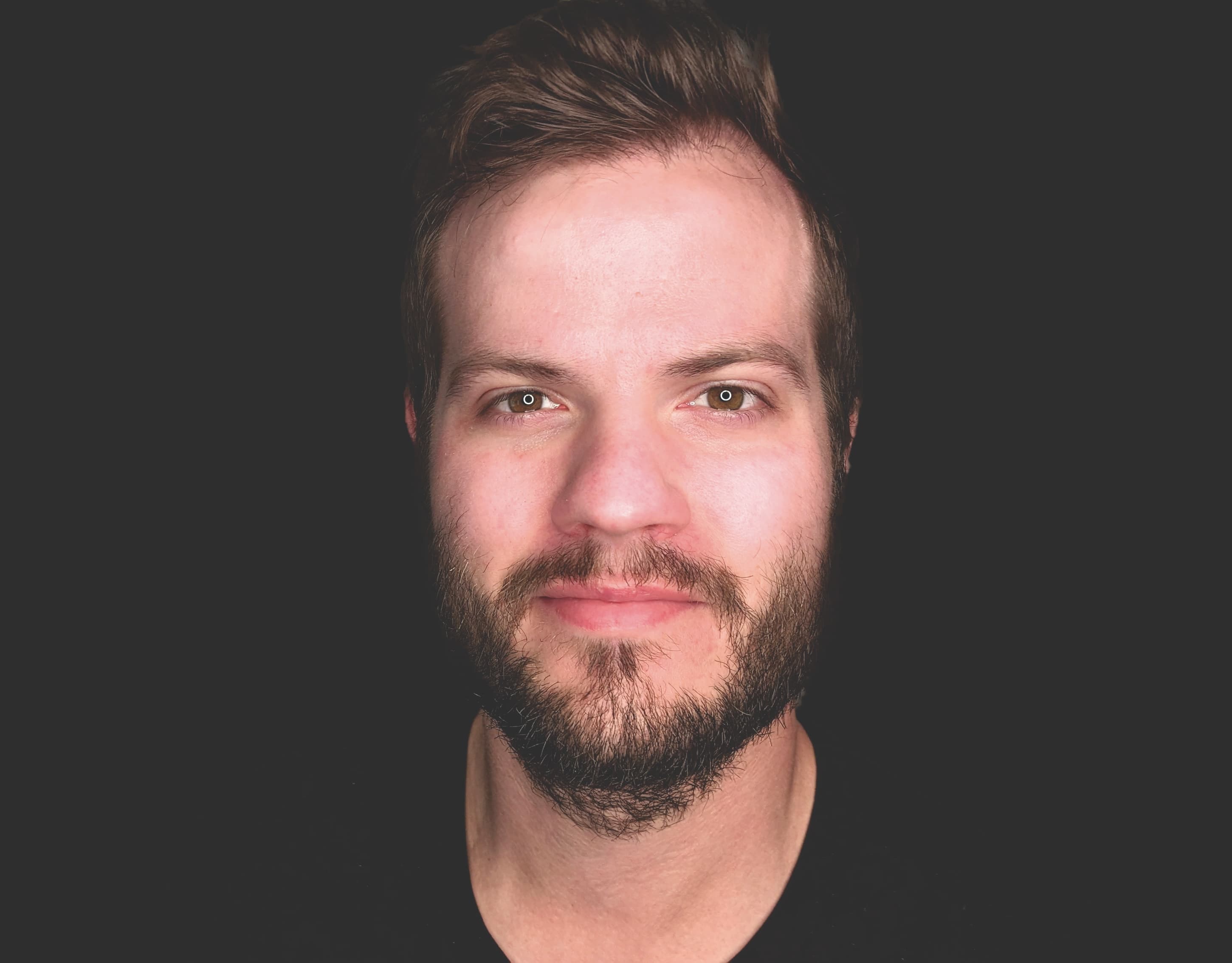
December 17, 2023
•Last updated December 19, 2023
Best Practices for Naming Models in Ruby on Rails
In Ruby on Rails, naming models is more than just a convention. It's about clarity, efficiency, and ensuring your app is easy to manage. Here's a guide to help you name your models like the pros.
P.S. I created a handy guide at https://web-crunch.com/naming-models. Bookmark it if you need a refresher every once in a while!
Singular and Capitalized
Model names should be singular and capitalized. Rails uses this convention to look for the corresponding pluralized table name in the database.
# Good
class Product < ApplicationRecord
end
# Avoid
class Products < ApplicationRecord
end
Keep it descriptive and clear
Names should be self-explanatory. Don't forget to avoid abbreviations unless they are well-known.
# Good
class ShoppingCart < ApplicationRecord
end
# Avoid
class SCart < ApplicationRecord
end
Organize with namespaces
For complex apps, namespaces help in organizing models. Use modules to group related models.
# Good
module Inventory
class Item < ApplicationRecord
end
end
# Usage
@item = Inventory::Item.new
Intuitive Associations
Keep it intuitive. If a User
has many articles
, the association should reflect that.
class User < ApplicationRecord
has_many :articles
end
Acronyms and Initialisms
If you're using acronyms, keep them uppercase.
# Good
class HTTPRequest < ApplicationRecord
end
# Avoid
class HttpRequest < ApplicationRecord
end
Avoid Reserved Words
Some words are reserved in Rails and should be avoided as model names (e.g., Attribute
, Error
).
Context-Specific Naming
If your app has models that only make sense within a specific context, name them accordingly.
# Good for a school management app
class GradeReport < ApplicationRecord
end
# Good for an e-commerce app
class PaymentGateway < ApplicationRecord
end
Avoid Ambiguity
Names should be unambiguous, even if they end up being longer.
# Good
class SubscriptionPayment < ApplicationRecord
end
# Avoid
class Payment < ApplicationRecord
# This could be ambiguous in an app dealing with multiple payment types
end
Composite Names
For models representing a combination of entities, use clear composite names.
# Good
class UserSubscriptionHistory < ApplicationRecord
end
# Avoid
class UserHistory < ApplicationRecord
# This is vague about what history it refers to
end
Polymorphic Associations
In polymorphic associations, choose names that clearly indicate their versatile nature.
class Picture < ApplicationRecord
belongs_to :imageable, polymorphic: true
end
class Employee < ApplicationRecord
has_many :pictures, as: :imageable
end
class Product < ApplicationRecord
has_many :pictures, as: :imageable
end
STI (Single Table Inheritance)
The model name should indicate its role if you use Single Table Inheritance.
# Good
class Vehicle < ApplicationRecord
end
class Car < Vehicle
end
class Motorcycle < Vehicle
end
Conclusion
Remember, well-named models make your code cleaner and more accessible for others (or future you) to understand and maintain. I hope these examples add more depth to your understanding! Look for more articles like this to come. In the meantime, check out some related content:
- A Beginner's Guide to Ruby on Rails Callbacks
- Understanding Active Record Callbacks
- Understanding Active Record Associations
- Understanding Active Record Migrations
- Understanding Ruby on Rails ActiveRecord Validations
P.P.S. Reminder! I created a handy guide at https://web-crunch.com/naming-models. Bookmark it if you need a refresher every once in a while!
Categories
Collection
Part of the Ruby on Rails collection
Products and courses
-
Hello Hotwire
A course on Hotwire + Ruby on Rails.
-
Hello Rails
A course for newcomers to Ruby on Rails.
-
Rails UI
UI templates and components for Rails.